First create Windows Form Application give any project name. Here I will give the project name as LINQtoObject. Then Design the Form using one Button and One textbox like in the picture below.
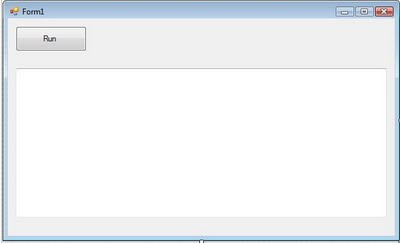
Now Do the following coding part

How this code work
First step is to reference the System.Linq namespace, which is done automatically by Visual Studio 2008 when you create project.
Next step is to create some data, which is done in this tutorial declaring the a array named names.
Next part of the program is writing the LINQ query.
var queryResults =from n in names where n.StartsWith(“S”) select n;
This is very similar to SQL commands. Isn't it? So if you are familiar with SQL this will very easy to understand for you.
Now Click on the debug button and click the Run button .The you will see the following result inside the textbox.
Query Results:
================
Sandaruwan
Sameera
Sampath
Sanjeewa
Sachith
Understanding the Coding part
Above LINQ query has four parts. The result variable declaration beginning with var , which is assigned using a query expression consisting of the from clause, the where clause, and the select cause. Let's look at each of these part one by one.
Declaring a Variable for result using var Keyword.
The LINQ query start by declaring a variable to hold the results of the query, which is usually done by declaring a variable with var keyword:
var queryResult=
Specify Data Source: from Clause
The next part of the LINQ query is the from clause, Which specifies the data you are querying.
from n in names
Specify Condition: where Clause
In this part of the LINQ query you specify the condition for your query using the where clause. Which look like this:
where n.StartsWith(“S”)
here I specify that the name string starts with the letter S, But you could specify anything else about the instead. For example , a length greater than 10( where n.Length>10) or containing a Q (where n.Contains("Q")).
Select Items: select Clause
Finally , the select clause specifies which items appear in the result set. The select clause look like this.
select n